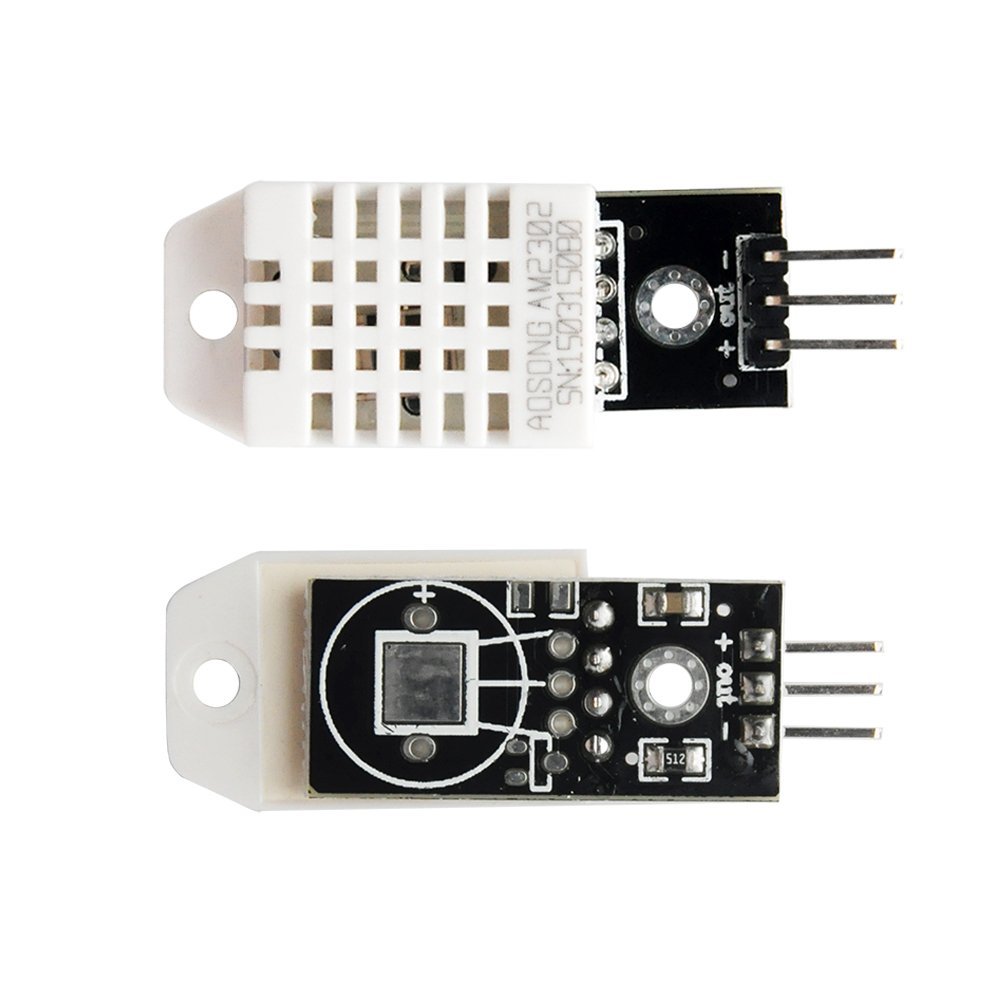
Authorized Online Retailer
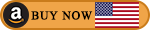
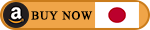
Description
DHT-22 (also known by RHT03) is a low-cost humidity and temperature sensor with a single wire digital interface. The sensor is calibrated and doesn’t require extra components, so you can get right to measuring relative humidity and temperature. DHT22 is more accurate and has more dynamic range than DHT11 sensors.
Technical Specifications
- 3.3-5.5V Input
- 1-1.5mA measuring current
- 40-50 uA standby current
- Humidity from 0-100% RH
- -40 – 80 degrees C temperature range
- +-2% RH accuracy
- +-0.5 degrees C
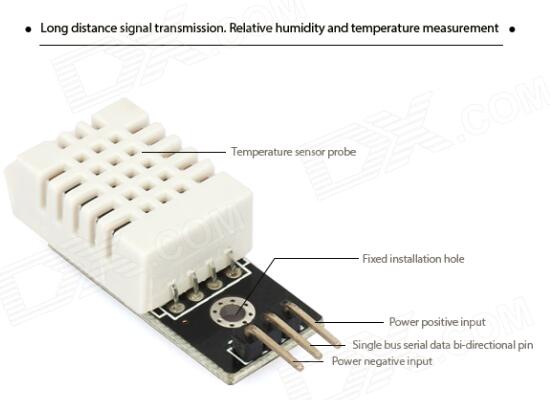
Documents and Downloads
Connection with Arduino
DHT22 |
Arduino |
GND |
GND |
VCC |
5V |
DATA |
D8 |
Source Code
First we need to include the DHT library which can be found from the Arduino official website, then define the pin number to which our sensor is connected and create a DHT object. In the setup section, we need to initiate the serial communication because we will use the serial monitor to print the results. Using the read22() function, we will read the data from the sensor and put the values of the temperature and the humidity into the t and h variables. If you use the DHT11 sensor, you will need to you the read11() function. At the end, we will print the temperature and the humidity values on the serial monitor.
- /* DHT11/ DHT22 Sensor Temperature and Humidity Tutorial
- * Program made by Dejan Nedelkovski,
- * www.HowToMechatronics.com
- */
- /*
- * You can find the DHT Library from Arduino official website
- * https://playground.arduino.cc/Main/DHTLib
- */
- #include
- #define dataPin 8 // Defines pin number to which the sensor is connected
- dht DHT; // Creats a DHT object
- void setup() {
- Serial.begin(9600);
- }
- void loop() {
- int readData = DHT.read22(dataPin); // Reads the data from the sensor
- float t = DHT.temperature; // Gets the values of the temperature
- float h = DHT.humidity; // Gets the values of the humidity
- // Printing the results on the serial monitor
- Serial.print(“Temperature = “);
- Serial.print(t);
- Serial.print(” *C “);
- Serial.print(” Humidity = “);
- Serial.print(h);
- Serial.println(” % “);
- delay(2000); // Delays 2 secods, as the DHT22 sampling rate is 0.5Hz
- }